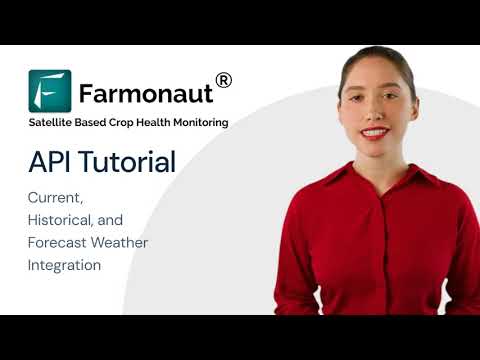
Weather data integration is becoming increasingly crucial for farmers and agricultural companies worldwide. By leveraging advanced weather APIs, these organizations can make informed decisions, improve crop yields, and manage resources more efficiently. In this comprehensive tutorial, we’ll explore how to integrate weather data using the Farmonaut API, a powerful tool for accessing current, historical, and forecast weather information.
🌤️ Overview of Weather Data Endpoints
The Farmonaut API offers several endpoints for retrieving different types of weather data. Let’s explore each of these endpoints and how to use them effectively in your weather API javascript implementation.
🌡️ Retrieving Present Weather Data
To get current weather information, use the “Get Present Weather” endpoint. This weather API js function requires only the user ID (uid) and field ID as parameters:
// Example weather API javascript code fetch('https://api.farmonaut.com/v1/get-present-weather', { method: 'POST', body: JSON.stringify({ uid: 'your-user-id', fieldId: 'your-field-id' }) }) .then(response => response.json()) .then(data => console.log(data));
This weather API test will return comprehensive current weather data, including temperature, humidity, wind speed, and more.
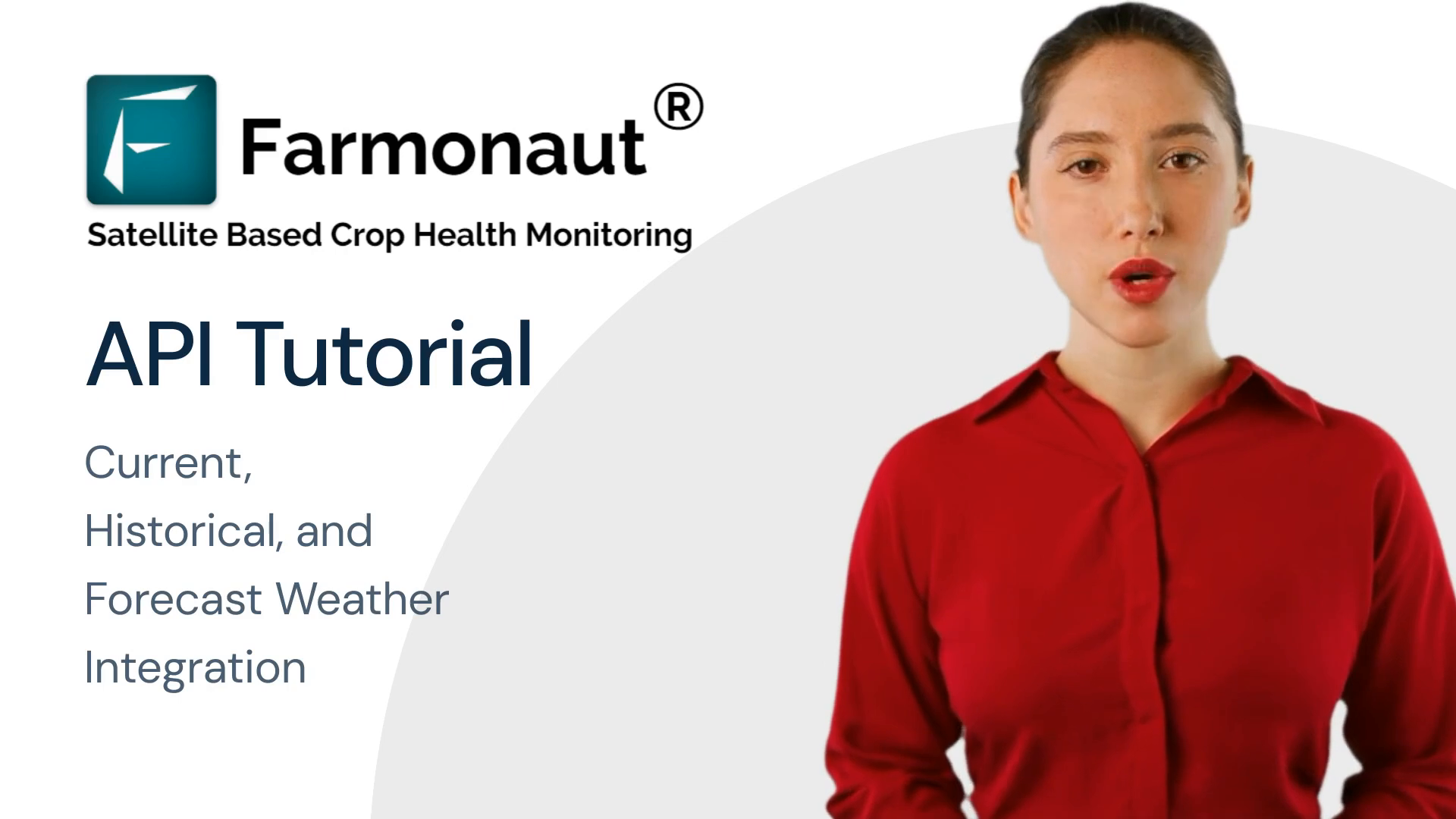
📅 Getting Historical Weather Data
For past weather information, use the “Get Historical Field Weather” endpoint. This weather database API call requires the uid, field ID, and the number of days for which you want historical data:
// Example weather API testing code for historical data fetch('https://api.farmonaut.com/v1/get-historical-field-weather', { method: 'POST', body: JSON.stringify({ uid: 'your-user-id', fieldId: 'your-field-id', days: 5 }) }) .then(response => response.json()) .then(data => console.log(data));
This weather data API request will provide historical weather information for the specified number of days.
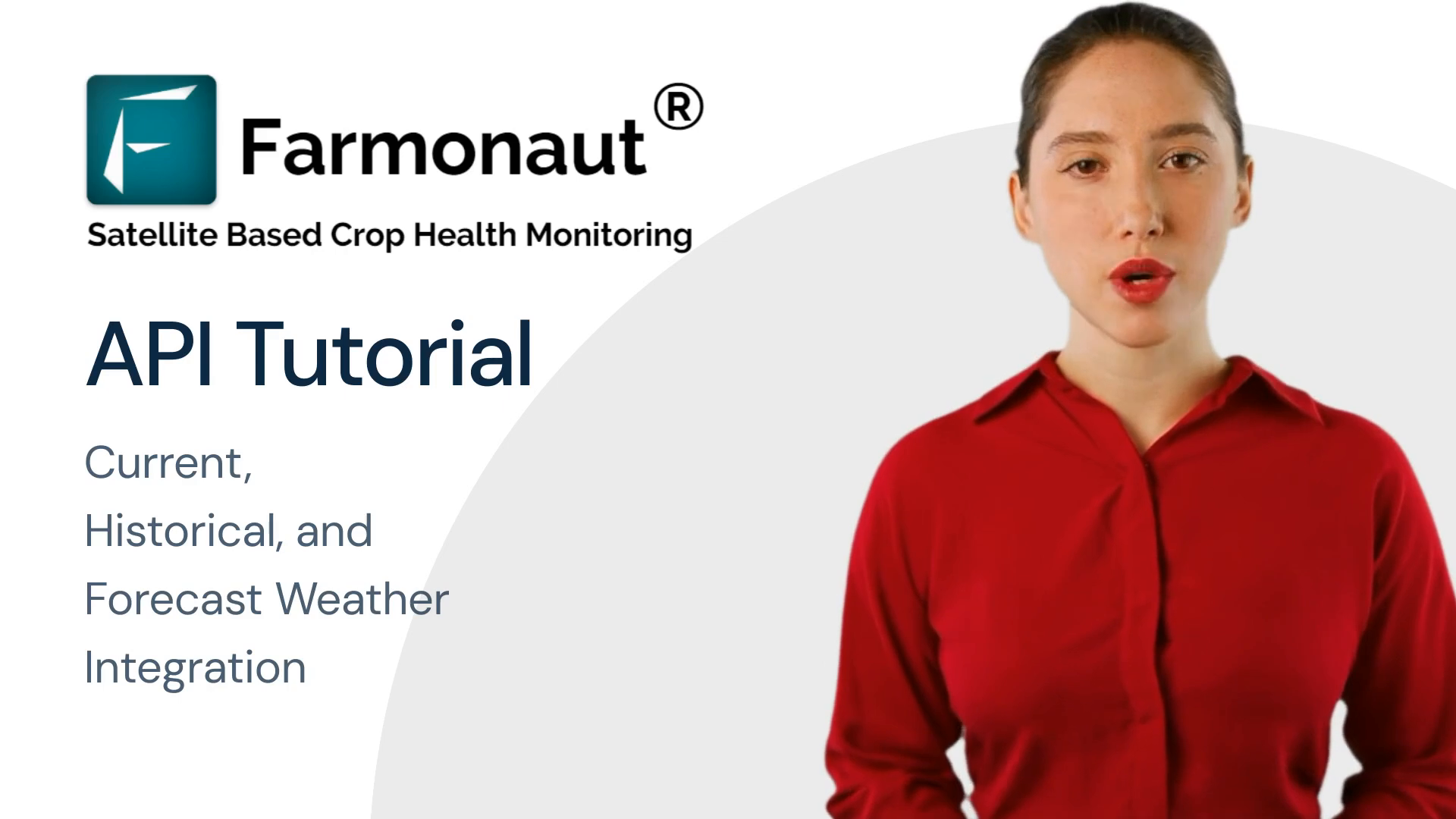
🔮 Fetching Weather Forecast Data
To retrieve future weather predictions, use the “Get Forecast Weather” endpoint. This weather forecast API call requires the uid and field ID:
// Example weather API js code for forecast data fetch('https://api.farmonaut.com/v1/get-forecast-weather', { method: 'POST', body: JSON.stringify({ uid: 'your-user-id', fieldId: 'your-field-id' }) }) .then(response => response.json()) .then(data => console.log(data));
This weather API testing request will return detailed forecast information, including daily predictions for temperature, humidity, wind speed, and more.
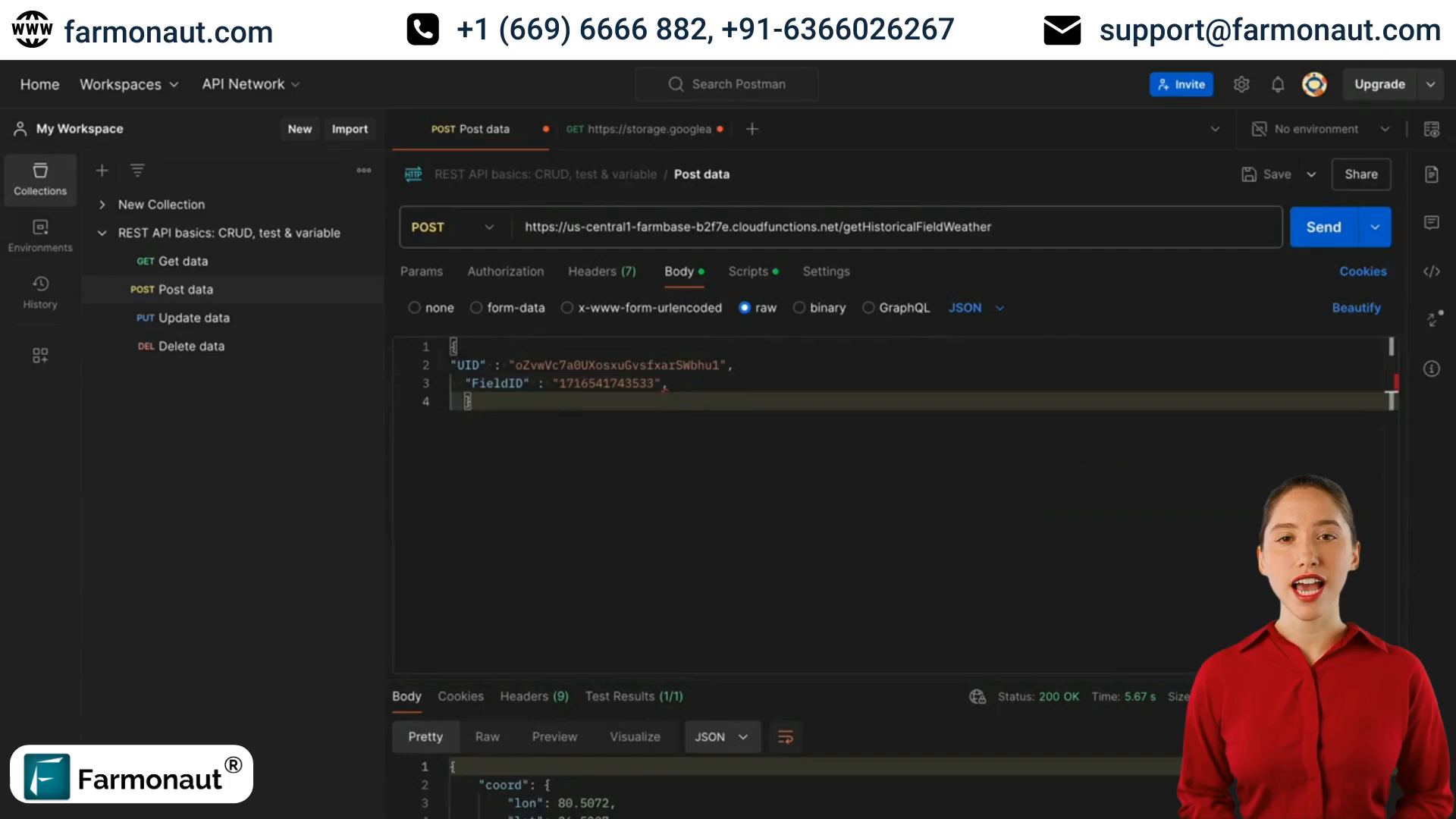
🌎 Retrieving Forecast Data by Latitude and Longitude
For location-specific forecasts, use the “Get Forecast Weather from Lat Long” endpoint. This weather API test requires the uid, latitude, and longitude:
// Example weather data API code for location-specific forecast fetch('https://api.farmonaut.com/v1/get-forecast-weather-from-lat-long', { method: 'POST', body: JSON.stringify({ uid: 'your-user-id', latitude: 12.3456, longitude: 78.9012 }) }) .then(response => response.json()) .then(data => console.log(data));
This weather forecast API call will provide detailed weather predictions for the specified coordinates.
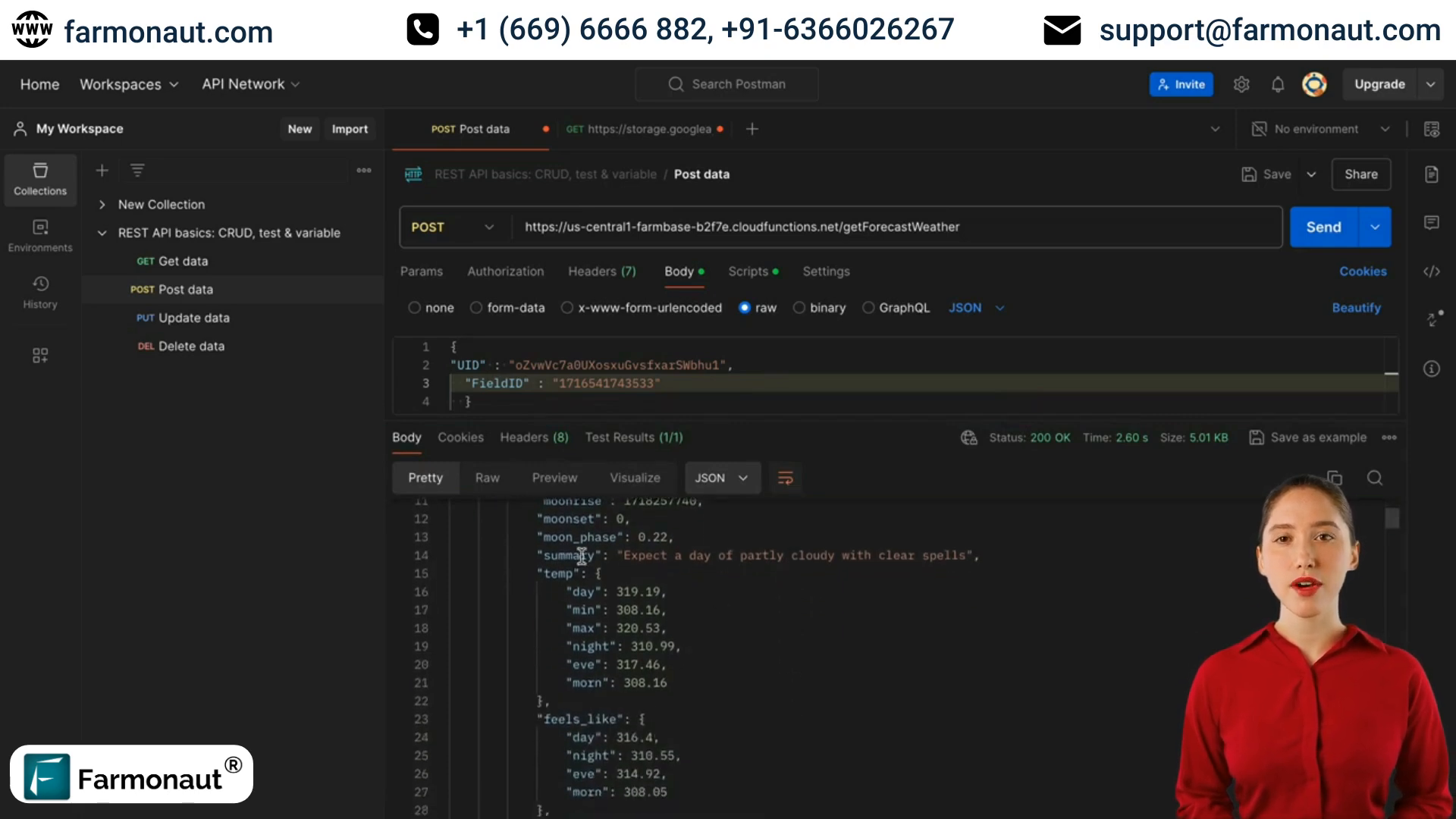
📊 Generating Weather Trend Graphs
The Farmonaut API also offers a unique feature to generate visual representations of weather trends. Use the “Get Past Weather Graph” endpoint to retrieve URLs for weather trend graphs:
// Example weather API javascript code for generating trend graphs fetch('https://api.farmonaut.com/v1/get-past-weather-graph', { method: 'POST', body: JSON.stringify({ fieldId: 'your-field-id', timestamp: 'desired-timestamp' }) }) .then(response => response.json()) .then(data => console.log(data.graphUrl));
This weather database API request will return a URL that can be used to display or download weather trend graphs.
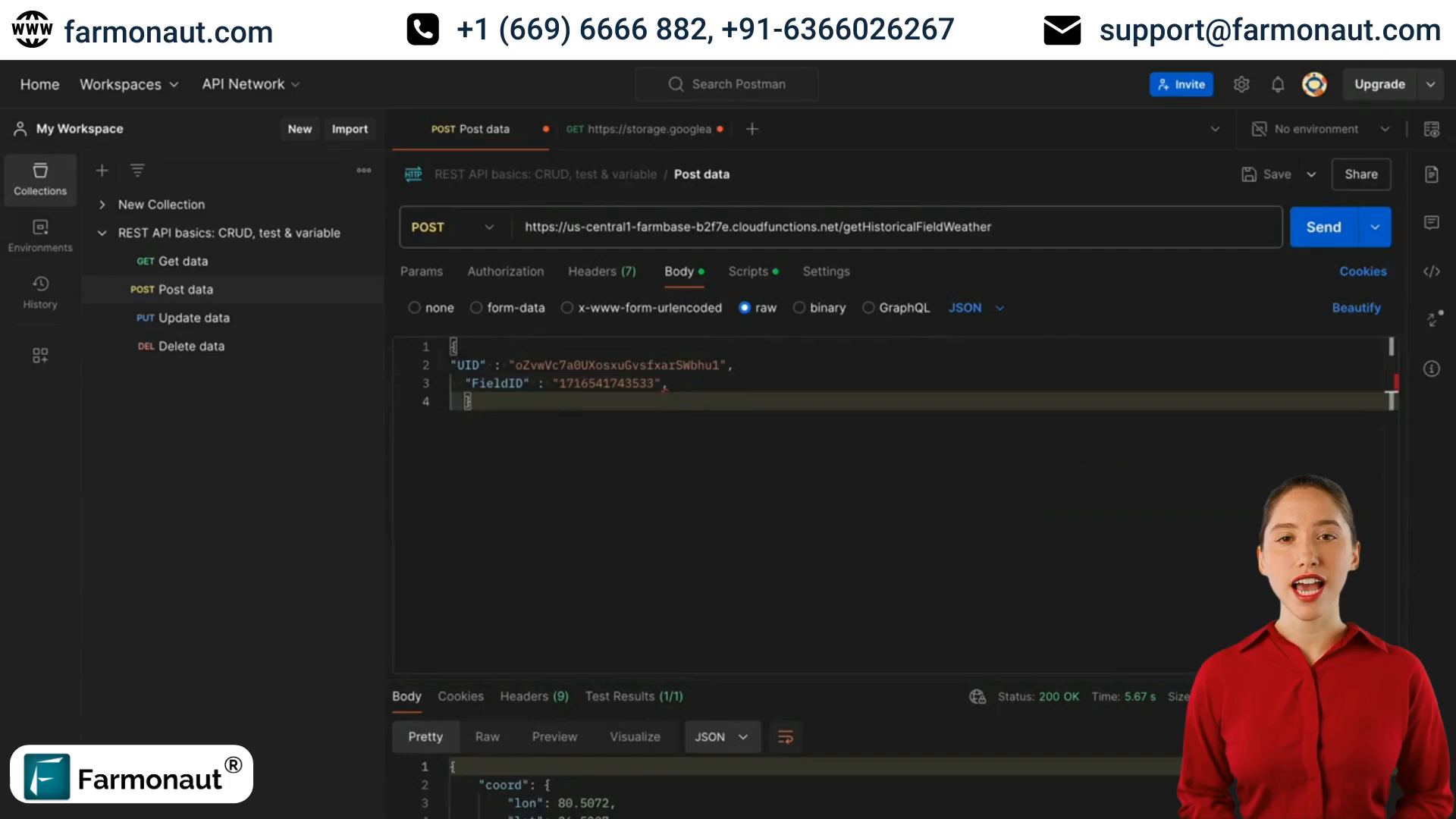
🎓 Conclusion
By integrating the Farmonaut API into your applications, you can access a wealth of weather data to enhance agricultural decision-making. This comprehensive weather data API allows you to retrieve current conditions, historical data, and future forecasts, as well as generate visual representations of weather trends.
Remember to conduct thorough weather API testing to ensure smooth integration and optimal performance in your applications. With these powerful tools at your disposal, you can significantly improve agricultural operations and yield management.
❓ Frequently Asked Questions
Q: What types of weather data can I access through the Farmonaut API?
A: The Farmonaut API provides access to current weather conditions, historical weather data, weather forecasts, and even weather trend graphs.
Q: How do I authenticate my requests to the Farmonaut API?
A: Most endpoints require a user ID (uid) for authentication. Ensure you include this in your API requests.
Q: Can I get weather data for a specific location without a field ID?
A: Yes, you can use the “Get Forecast Weather from Lat Long” endpoint to retrieve weather data for any location using its latitude and longitude coordinates.
Q: How far into the future can I get weather forecast data?
A: The forecast data typically covers several days into the future, but the exact duration may vary. Check the API documentation for the most up-to-date information.
Q: Are there any rate limits for using the Farmonaut API?
A: Rate limits may apply depending on your subscription plan. Refer to the API documentation or contact Farmonaut support for specific details about rate limits.